C Library
PHP Module
Perl Module
Ruby Library
Python Library
.NET Component
Java Component
Apache Module
Nginx Module
Node.js Module
HTTP Module
Go Package
D Library
Lua Package
OpenResty Package
Splunk Add-on
Erlang Module
Haskell Package
Kotlin Module
Scala Library
Apache Kafka Transform
Deno Module
R Package
IP2Proxy .NET Component
This .NET component supports all IP2Proxy™ BIN database products to query an IP address if it is being used as virtual private networks (VPN), open proxies, web proxies, Tor exits, data center & web hosting ranges (DCH), search engine robots (SES), residential proxies (RES), consumer privacy networks (CPN), and enterprise private networks (EPN). It has been optimized for speed and memory utilization.
Installation
To install IP2Proxy, run the following command in the Package Manager Console
PM> Install-Package IP2Proxy
Sample Codes
Imports System.IO
Module TestIP2Proxy
Sub Main()
Dim proxy As New IP2Proxy.Component
Dim all As IP2Proxy.ProxyResult
Dim isproxy As Integer
Dim proxytype As String
Dim countryshort As String
Dim countrylong As String
Dim region As String
Dim city As String
Dim isp As String
Dim domain As String
Dim usagetype As String
Dim asn As String
Dim [as] As String
Dim lastseen As String
Dim threat As String
Dim provider As String
Dim ip As String = "46.101.133.66"
If proxy.Open("C:\data\IP2PROXY-IP-PROXYTYPE-COUNTRY-REGION-CITY-ISP-DOMAIN-USAGETYPE-ASN-LASTSEEN-THREAT-RESIDENTIAL-PROVIDER.BIN", IP2Proxy.Component.IOModes.IP2PROXY_MEMORY_MAPPED) = 0 Then
Console.WriteLine("GetModuleVersion: " & proxy.GetModuleVersion())
Console.WriteLine("GetPackageVersion: " & proxy.GetPackageVersion())
Console.WriteLine("GetDatabaseVersion: " & proxy.GetDatabaseVersion())
' reading all available fields
all = proxy.GetAll(ip)
Console.WriteLine("Is_Proxy: " & all.Is_Proxy.ToString())
Console.WriteLine("Proxy_Type: " & all.Proxy_Type)
Console.WriteLine("Country_Short: " & all.Country_Short)
Console.WriteLine("Country_Long: " & all.Country_Long)
Console.WriteLine("Region: " & all.Region)
Console.WriteLine("City: " & all.City)
Console.WriteLine("ISP: " & all.ISP)
Console.WriteLine("Domain: " & all.Domain)
Console.WriteLine("Usage_Type: " & all.Usage_Type)
Console.WriteLine("ASN: " & all.ASN)
Console.WriteLine("AS: " & all.AS)
Console.WriteLine("Last_Seen: " & all.Last_Seen)
Console.WriteLine("Threat: " & all.Threat)
Console.WriteLine("Provider: " & all.Provider)
' reading individual fields
isproxy = proxy.IsProxy(ip)
Console.WriteLine("Is_Proxy: " & isproxy.ToString())
proxytype = proxy.GetProxyType(ip)
Console.WriteLine("Proxy_Type: " & proxytype)
countryshort = proxy.GetCountryShort(ip)
Console.WriteLine("Country_Short: " & countryshort)
countrylong = proxy.GetCountryLong(ip)
Console.WriteLine("Country_Long: " & countrylong)
region = proxy.GetRegion(ip)
Console.WriteLine("Region: " & region)
city = proxy.GetCity(ip)
Console.WriteLine("City: " & city)
isp = proxy.GetISP(ip)
Console.WriteLine("ISP: " & isp)
domain = proxy.GetDomain(ip)
Console.WriteLine("Domain: " & domain)
usagetype = proxy.GetUsageType(ip)
Console.WriteLine("Usage_Type: " & usagetype)
asn = proxy.GetASN(ip)
Console.WriteLine("ASN: " & asn)
[as] = proxy.GetAS(ip)
Console.WriteLine("AS: " & [as])
lastseen = proxy.GetLastSeen(ip)
Console.WriteLine("Last_Seen: " & lastseen)
threat = proxy.GetThreat(ip)
Console.WriteLine("Threat: " & threat)
provider = proxy.GetProvider(ip)
Console.WriteLine("Provider: " & provider)
Else
Console.WriteLine("Error reading BIN file.")
End If
proxy.Close()
End Sub
End Module
namespace TestIP2ProxyComponent
{
class TestIP2Proxy
{
static void Main(string[] args)
{
IP2Proxy.Component proxy = new IP2Proxy.Component();
IP2Proxy.ProxyResult all;
int isproxy;
string proxytype;
string countryshort;
string countrylong;
string region;
string city;
string isp;
string domain;
string usagetype;
string asn;
string @as;
string lastseen;
string threat;
string provider;
string ip = "221.121.146.0";
if (proxy.Open("C:\\data\\IP2PROXY-IP-PROXYTYPE-COUNTRY-REGION-CITY-ISP-DOMAIN-USAGETYPE-ASN-LASTSEEN-THREAT-RESIDENTIAL-PROVIDER.BIN", IP2Proxy.Component.IOModes.IP2PROXY_MEMORY_MAPPED) == 0)
{
System.Console.WriteLine("GetModuleVersion: " + proxy.GetModuleVersion());
System.Console.WriteLine("GetPackageVersion: " + proxy.GetPackageVersion());
System.Console.WriteLine("GetDatabaseVersion: " + proxy.GetDatabaseVersion());
// reading all available fields
all = proxy.GetAll(ip);
System.Console.WriteLine("Is_Proxy: " + all.Is_Proxy.ToString());
System.Console.WriteLine("Proxy_Type: " + all.Proxy_Type);
System.Console.WriteLine("Country_Short: " + all.Country_Short);
System.Console.WriteLine("Country_Long: " + all.Country_Long);
System.Console.WriteLine("Region: " + all.Region);
System.Console.WriteLine("City: " + all.City);
System.Console.WriteLine("ISP: " + all.ISP);
System.Console.WriteLine("Domain: " + all.Domain);
System.Console.WriteLine("Usage_Type: " + all.Usage_Type);
System.Console.WriteLine("ASN: " + all.ASN);
System.Console.WriteLine("AS: " + all.AS);
System.Console.WriteLine("Last_Seen: " + all.Last_Seen);
System.Console.WriteLine("Threat: " + all.Threat);
System.Console.WriteLine("Provider: " + all.Provider);
// reading individual fields
isproxy = proxy.IsProxy(ip);
System.Console.WriteLine("Is_Proxy: " + isproxy.ToString());
proxytype = proxy.GetProxyType(ip);
System.Console.WriteLine("Proxy_Type: " + proxytype);
countryshort = proxy.GetCountryShort(ip);
System.Console.WriteLine("Country_Short: " + countryshort);
countrylong = proxy.GetCountryLong(ip);
System.Console.WriteLine("Country_Long: " + countrylong);
region = proxy.GetRegion(ip);
System.Console.WriteLine("Region: " + region);
city = proxy.GetCity(ip);
System.Console.WriteLine("City: " + city);
isp = proxy.GetISP(ip);
System.Console.WriteLine("ISP: " + isp);
domain = proxy.GetDomain(ip);
System.Console.WriteLine("Domain: " + domain);
usagetype = proxy.GetUsageType(ip);
System.Console.WriteLine("Usage_Type: " + usagetype);
asn = proxy.GetASN(ip);
System.Console.WriteLine("ASN: " + asn);
@as = proxy.GetAS(ip);
System.Console.WriteLine("AS: " + @as);
lastseen = proxy.GetLastSeen(ip);
System.Console.WriteLine("Last_Seen: " + lastseen);
threat = proxy.GetThreat(ip);
System.Console.WriteLine("Threat: " + threat);
provider = proxy.GetProvider(ip);
System.Console.WriteLine("Provider: " + provider);
}
else
{
System.Console.WriteLine("Error reading BIN file.");
}
proxy.Close();
}
}
}
Sample IP2Proxy Databases (BIN)
Sample Packages - BIN File | IPv4 + IPv6 |
---|---|
IP2Proxy PX1 | Download (20.69 MB) |
IP2Proxy PX2 | Download (23.17 MB) |
IP2Proxy PX3 | Download (27.55 MB) |
IP2Proxy PX4 | Download (32.29 MB) |
IP2Proxy PX5 | Download (33.72 MB) |
IP2Proxy PX6 | Download (34.01 MB) |
IP2Proxy PX7 | Download (36.69 MB) |
IP2Proxy PX8 | Download (37.84 MB) |
IP2Proxy PX9 | Download (37.69 MB) |
IP2Proxy PX10 | Download (45.43 MB) |
IP2Proxy PX11 | Download (46.34 MB) |
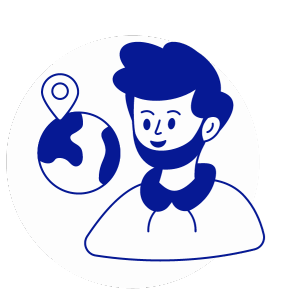
Discover Your User Locations
Retrieve geolocation data for FREE now!